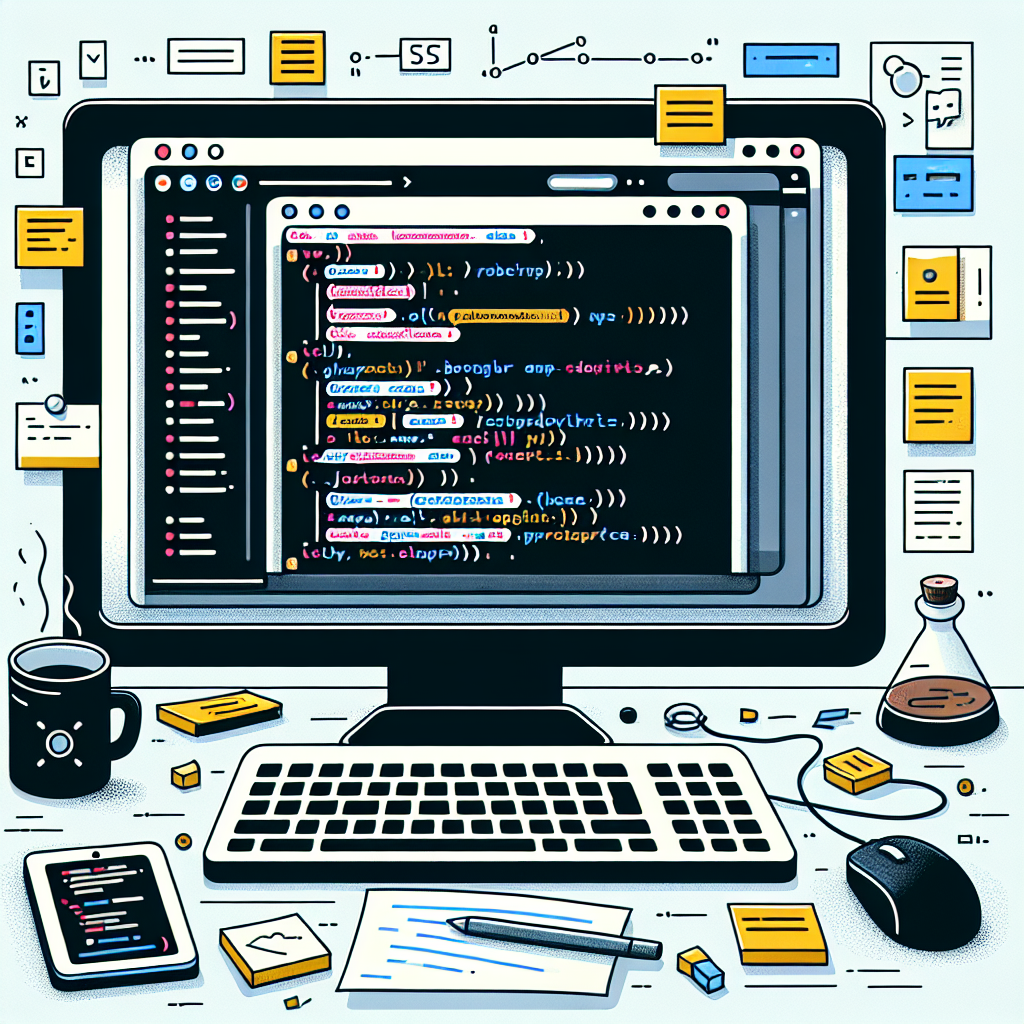
Handling Browser Close Events with JavaScript
Welcome back to another episode of “Continuous Improvement”. I’m your host, Victor, and today we’ll be discussing an important aspect of user experience on the web - preventing accidental page exits. Have you ever been in a situation where you were filling out a form or making a payment, and accidentally closed your browser, losing all your progress? Well, we have a solution for you.
In this episode, we’ll dive deep into the implementation of a confirmation dialog using the beforeunload
event in JavaScript. This will help you prompt users with a warning before they close their browsers, ensuring they are aware of their unsaved changes. So, let’s get started!
[BACKGROUND FADES]
First, let’s take a look at what the confirmation dialog actually looks like. In different browsers, it can vary slightly in appearance. In Chrome, for example, it may look like this. [DESCRIBING CHROME DIALOG]
And in Firefox, it may appear slightly different. [DESCRIBING FIREFOX DIALOG]
[BACKGROUND FADES]
So, how can you implement this dialog on your web page? It’s actually quite simple. Just add the following code to your JavaScript file:
window.addEventListener('beforeunload', (event) => {
// Cancel the event as specified by the standard.
event.preventDefault();
// Chrome requires returnValue to be set.
event.returnValue = '';
});
By adding this event listener, you’re informing the browser to trigger the confirmation dialog when the user attempts to close the browser, refresh the page, or click the back button. The event.preventDefault()
cancels the event, ensuring the dialog is shown, and the event.returnValue = ''
satisfies Chrome’s requirements.
[BACKGROUND FADES]
It’s important to note that the beforeunload
event will only trigger if the user has interacted with the page in some way. If they haven’t, the event won’t activate. Once you’ve implemented this functionality, the confirmation dialog will keep users from inadvertently leaving the page without saving their changes or completing their transaction.
[BACKGROUND FADES]
But what if you want to remove the confirmation dialog at some point? Maybe after the user has saved the form or completed the payment. Well, you can easily do that too. Just use the following code:
window.removeEventListener('beforeunload', callback);
This line of code will remove the event listener, so the confirmation dialog no longer appears when attempting to leave the page. However, remember to replace callback
with the actual function or arrow function you used in the addEventListener
method.
[BACKGROUND FADES]
It’s worth mentioning that the purpose of this confirmation dialog is to remind users to save their changes before leaving, and it doesn’t provide any way to determine whether the user chose to stay or leave the page. So keep that in mind while implementing it in your project.
[BACKGROUND FADES]
And that’s a wrap for today’s episode of “Continuous Improvement”. We hope you found this topic helpful in enhancing user experience on your website. Remember, implementing a confirmation dialog using the beforeunload
event can prevent users from accidentally closing their browsers and losing their progress. For more detailed information and additional resources, you can check out the MDN Web Docs.
Thank you for listening to “Continuous Improvement”. I’m Victor, your host, and I’ll catch you in the next episode. Until then, happy coding!