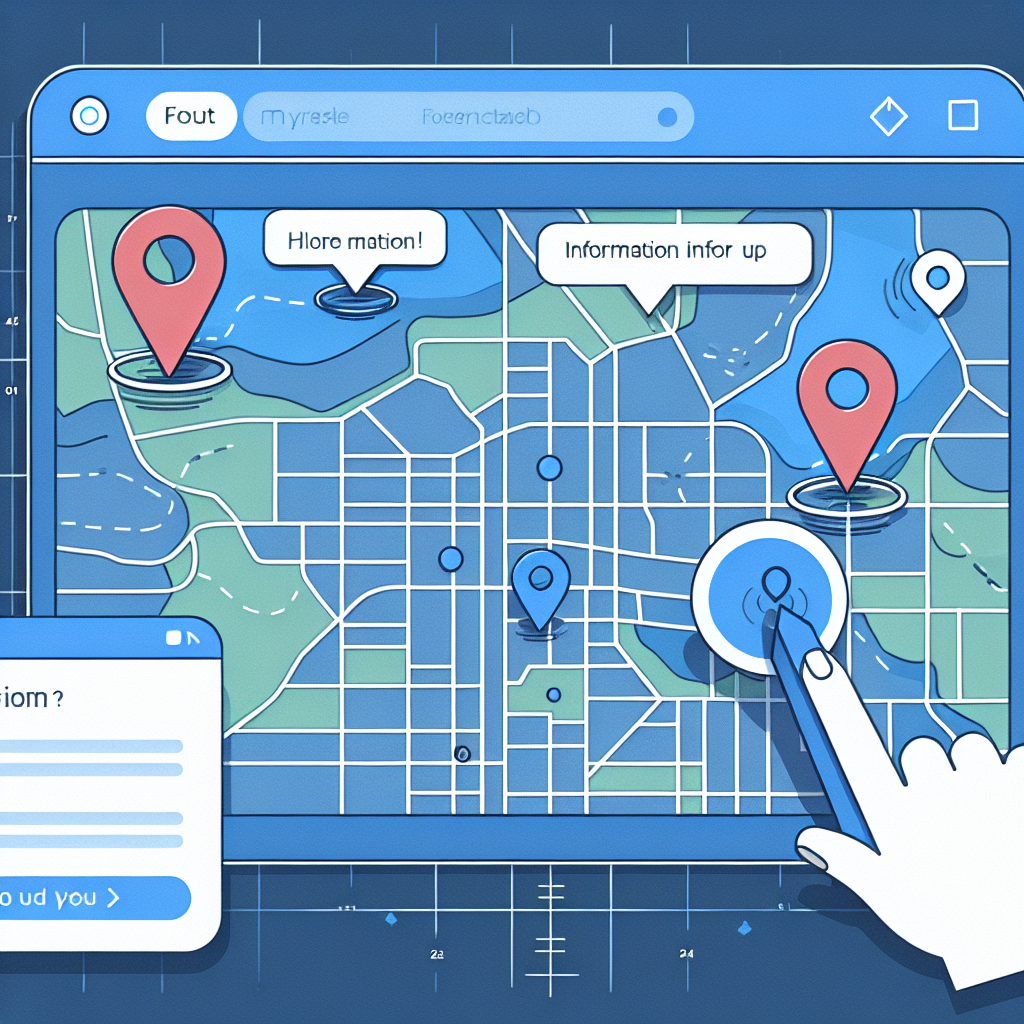
Setting Up a Proxy Server with Express
The Problem:
Welcome to “Continuous Improvement,” the podcast where we explore practical solutions to everyday problems. I’m your host, Victor. Today, we’re going to talk about a common issue when working with APIs and how to overcome it.
Imagine you’re working on a project that uses BreweryDB. You’re trying to load some data from the API, but suddenly, you encounter a problem. The API doesn’t support JSONP, leading to a CORS issue when you attempt to fetch data directly using Angular. Frustrating, right?
But fear not! I’ve got a solution for you. In this episode, I’ll guide you step-by-step on how to set up an intermediate proxy using Node.js and Express. This will allow you to avoid exposing your API key and resolve the CORS issue.
Let’s dive into the solution.
First, we need to install Express and Request. Open up your terminal and type in:
npm install express --save
npm install request --save
After the installations are complete, it’s time to create a server.js
file. In this file, we’ll set up the proxy using Node.js and Express. Make sure you have it ready before moving on to the next step.
So, let’s set up the route. Replace API_KEY
with your actual API key. This step is crucial to ensure proper authentication.
Inside your server.js
file, add the following code:
var express = require('express');
var request = require('request');
var app = express();
app.get('/api', function(req, res) {
request('https://api.brewerydb.com/v2/?key=' + API_KEY, function (error, response, body) {
if (!error && response.statusCode === 200) {
console.log(body);
res.send(body);
}
});
});
Great! Now that we have the route set up, let’s move on to setting up the port.
In your server.js
file, add the following code:
app.listen(3000);
console.log('Server running on port %d', 3000);
Superb! You’re almost there. Now it’s time to start the server. Open your terminal, navigate to the location of your server.js
file, and enter the following command:
node server.js
Congratulations! You’ve successfully set up the intermediate proxy using Node.js and Express.
It’s time to test your proxy. Open your browser and type in http://localhost:3000/api. If everything goes well, you should be able to see the JSON object and even log it in your browser console.
And that’s it! You’ve overcome the CORS issue by setting up an intermediate proxy. Now you can fetch data from the BreweryDB API without any problems.
If you encounter any difficulties or have any questions, please feel free to send me an email at victorleungtw@gmail.com. I’ll be more than happy to assist you.
Thank you for tuning in to this episode of “Continuous Improvement.” I hope you found the solution helpful and can apply it to your own projects. Don’t forget to subscribe to our podcast for more practical solutions to everyday problems. Until next time, keep improving.