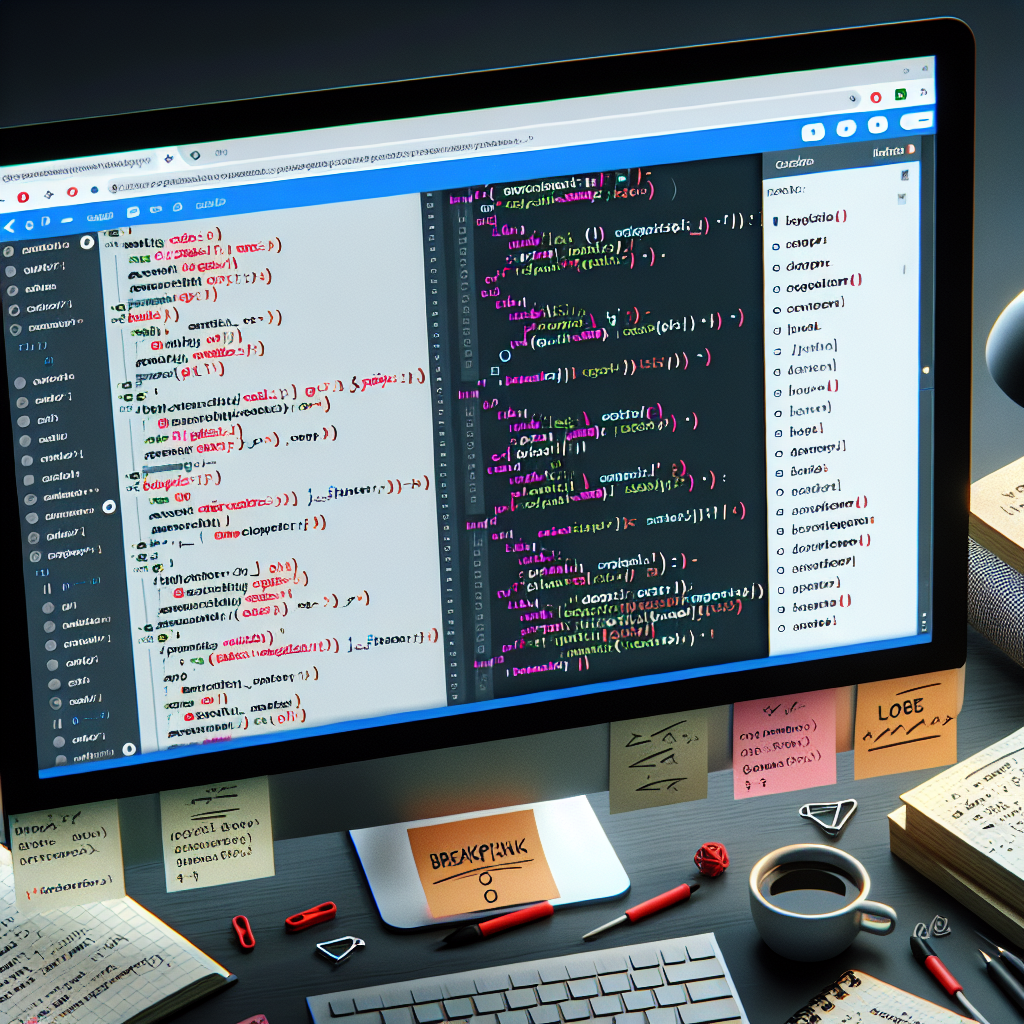
Debugging PHP Code in the Browser
In JavaScript, you can use console.log('whatever')
for troubleshooting directly in your browser. However, when working with PHP, a little trick is required to accomplish the same thing. Here are the steps:
-
Create a function named
debug_to_console
to handle the output to the console. Add this code to your PHP file:function debug_to_console($data) { $output = $data; if (is_array($output)) { $output = implode(',', $output); } echo "<script>console.log('Debug Objects: " . $output . "');</script>"; }
-
On the line where you need to output to the console, insert the following code:
debug_to_console("Test");
-
If you need to debug an object, you can log it like this:
debug_to_console(json_encode($foo));
After following these steps, open your browser’s developer tools. You should be able to see the PHP object displayed in the console using console.log
.